Tech Blog
By Ghaffar Khan
Monday, May 11, 2009
Sunday, May 3, 2009
Show your data on Google map using C# and JavaScript
Almost every one of us who use internet has seen the Google map, Google earth and Google street view etc. Good thing is that Google also share its products in a manner that you can customize them according to your needs. In this article I am going to share a simple technique to show your data on Google map.
Background
Previously I wrote an article on how to count the visits and visitors on your website and save the data in a table including the IPs of the visitors. Then one day I visited a site that was offering to show the similar data on Google map. I started to discover how I can do that if I have a database of IPs. I found that Google share some Maps APIs that offer lot of functionalities including placing a marker on the map if you can provide its coordinate, means the Latitude and longitude of that point on the earth.
Now the only problem was that I had to translate the IP into its corresponding coordinates. I searched again and found some web services on net that can return the coordinate of an IP. That’s all I needed to know to achieve the Goal and now it was just a matter of implementation.
How to do that .. Using the Code
Assuming that you have a table in a database that has two columns for earth coordinates you want to plot on Google map as shown below.
If you have a table that has IPs like in my previous article, you can add two new columns in that table, and then use some web services to update the table for coordinates manually or you can write a small program to call such a web service to update your database automatically. Or may be you want to show your shipments destinations on the map, or you might be interested in showing the location of showrooms of your products around the world and you have a similar data for that. Possibilities are endless.
Now how to plot this data on Google map? Google provides some API methods that you can call in JavaScript from your website and supply the coordinates to place a marker on the map. First you need to add APIs reference in your HTML in header tag as;
<-script src="http://maps.google.com/maps?file=api&v=2&sensor=false&key=abcdefg" type="text/JavaScript"><-/script>
Note: You need to obtain a unique key for your site from Google before publishing your site. For test purpose on localhost you can use the above code as it’s. Also
Second; you have to place a div element in the page where you want to show the Google map, set its size etc according to your layout needs.
<-div id="map_canvas" style="width: 100%; height: 728px; margin-bottom: 2px;">
Third, you can create a Google map object by passing it div element where you want to show the map.
var map = new GMap2(document.getElementById('map_canvas'));
Also you need to tell what location on the map should be in the center of map, first you get the point from Longitude and Latitude values. For example if you want to see London in the center of the map you can use method as
GLatLng(51.5,-0.1167)
Now you can pass this point to setCenter method with zoom level of your map ranges from 1-20, I am using level 2 to see whole world in my map, increasing this number will zoom the map to your center location.
map.setCenter(new GLatLng(51.5,-0.1167), 2);
Then you can specify a location on the map for Google to place a marker at that location by calling addOverlay method with GMarker object as argument that takes the actual coordinates of the location. For example to place a marker on Toronto, you can call write the following line in script.
map.addOverlay(new GMarker(new GLatLng(43.6667,-79.4168)));
You can specify as many markers as you want. Now you are ready to see the map. Put them all together in a JavaScript function and call it onload event of the page. The complete script will be something like;
<-script type='text/JavaScript'>
function initialize() {
if (GBrowserIsCompatible()) {
var map = new Map2(document.getElementById('map_canvas'));
map.setCenter(new GLatLng(51.5,-0.1167), 2);
map.addOverlay(new Marker(new GLatLng(43.6667,-79.4168)));
map.setUIToDefault();
} }
You have to generate the JavaScript on the server for Google APIs and render it on the client by using an “asp:Literal” control . You also need to place a Div control on the page in which Google APIs can render a customized map in the browser and add reference to Google APIs script. So you only need two controls on your page a Literal and a Div. The complete HTML is shown below including Google API references for MAP.
<-html xmlns="http://www.w3.org/1999/xhtml">
<-head id="Head1" runat="server">
<-title>Your Data on Google Map
<-%--Google API reference--%>
<-script src="http://maps.google.com/maps? file=api&v=2&sensor=false&key=abcdefg" type="text/JavaScript">
<-/script>
<-/head>
<-form id="form1" runat="server">
<-asp:Panel ID="Panel1" runat="server">
<-%--Place holder to fill with JavaScript by server side code--%>
<-asp:Literal ID="js" runat="server">
<-%--Place for Google to show your MAP--%>
<-div id="map_canvas" style="width: 100%; height: 728px; margin-bottom: 2px;">
<-/div>
<-/asp:Panel>
<-/form>
<-/body>
<-/html>
Now how to create the script on server that can show all of your markers on map? Here is a method to do that, you need to pass it the table discussed above that has Longitude and Latitude values in two columns, for the points you want to mark on MAP and this method will generate the complete script with all map markers in the Literal control on the page.
private void BuildScript(DataTable tbl)
{
String Locations = "";
foreach (DataRow r in tbl.Rows)
{
// bypass empty rows
if (r["Latitude"].ToString().Trim().Length == 0)
continue;
string Latitude = r["Latitude"].ToString();
string Longitude = r["Longitude"].ToString();
// create a line of JavaScript for marker on map for this record
Locations += Environment.NewLine + " map.addOverlay(new GMarker(new GLatLng(" + Latitude + "," + Longitude + ")));";
}
// construct the final script
js.Text = @"<-script type='text/JavaScript'>
function initialize() {
if (GBrowserIsCompatible()) {
var map = new GMap2(document.getElementById('map_canvas'));
map.setCenter(new GLatLng(51.5,-0.1167), 2);
" + Locations + @"
map.setUIToDefault();
}
}
<-/script> ";
}
One you call this method in the .cs file of your ASP form, it will create a complete JavaScript for Google to create a map and marker on your page.
Click here to see a live demo of this article. That site shows a marker on map for each location from where any of my sites has been visited. If you have visited any of my sites you should see a marker on your city on the map. Here is a snap of that demo site.
I have created a sample project for this article that you can download by clicking here. It also has a sample access database in it. You can simply run this project in Microsoft Visual Studio 2008 without changing anything.
You can visit Google page about Maps APIs for more details about using and customizing Google Maps.
Sunday, April 12, 2009
Site statistics with PHP and MySQL
Last week I developed a module for my websites to keep record of visitors on any of my websites. Objective was to make such a module that can be hosted on one location and can be used in sites on different servers.
Main features of the module are;
- Count every visit on a page.
- Count unique visitors on the same page.
- Show the figures on the page.
Result of all above implantation is in the picture below that shows the statistics of this post. This is a real execution of the code, it records the visitors on this page. You can see the changes in numbers by refreshing this page.
Then I built another module to use the data generated by first module to create a graphical representation for the history of last thirty days.
This is also iThis is also integrated in all my blogs, in side panel. You might can see this in action in the side panel of this page.After completing all this, now I have data that can be used in many ways. I extended with another module that can show me the summary of visits on all of my sites as below.
Another graph I created to see the share of visitor each of my site is getting.
Another graph shows the detailed comparison of each site date wise number of visitors;
Technical Details:
I have written all the code in PHP to achieve this functionality and used MySql to manage its data. For graphs I used PHPGraphLib modules.
Counter:
Initially I used a PHP graphical hit counter to count the visits on my site, that stores the data in a file on the server. But I gradually changed it completely to store data in a MySql database, by keeping its graphical digits display techniques as it is. Plus I extended the same module to count both visits and unique visitors.
My Personal Web Site
Recently I decided to build a web site for m y personal stuff. Initially I had two goals in my mind. To organize and publish my contents on internet and to exercise some techniques on the same web site in development.I started with a traditional website lay out in simple HTML and CSS with static contents. Then turned it into a PHP website to handle multiple pages with same layout. Then I added some coding to make it little dynamic with expending and collapsing menus with PHP and AJAX the internal processing of my PHP coding was inspired by Mambo and Joomla UI for content management but it was completely my own written code different then any other CMS. Then started adding links to my contents on flickr and you tube etc. and then I came to know a whole new world of widgets and gadget to embed and share the web contents. I did by embedding different objects from different sources, like you tube, slide share and flickr. Here is the is version of my website after implementing all above click the image below to visit the site.
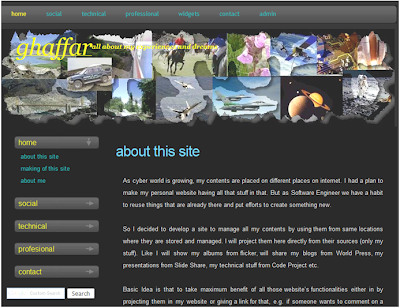
When I first saw iGoogle in 2006, I was inspired by the technology and it was my first motive to know about AJAX technology. I had in my mind to make my site something like that having dynamic and moveable contents. In last six months I did lot of research on this technique red many articles and work of some other people. Finally I started building my own dashboard for my web site. I used JQuery to achieve to achieve dynamic behavior. Plus I created a standard for widgets for my dashboard after observing modules in PageFlakes and IGoogle and made a widget library for my dashboard. It is all in action now. In the site, each window is an independent widget that can be minimized, expanded and rearranged by viewer. Each widget has its own content and functionality. In this way I can add lot of contents on a single page and viewer don’t have to leave a page to go to new page. Some widgets can even be maximized to full screen or can show themselves in larger floating window on the same page.
You can visit the live website by clicking on the image below;
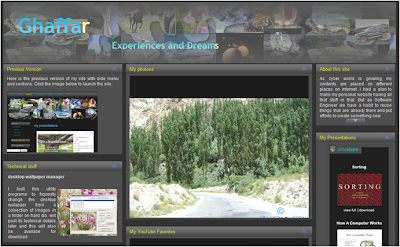
I will discuss some techniques I have applied in my site in my future blogs.
Ghaffar
Sunday, March 29, 2009
Computer Graphics Introduction
